Deep Copy and Shallow Copy in Python
Last Updated :
10 Dec, 2024
Improve
In Python, assignment statements create references to the same object rather than copying it. Python provides the copy module to create actual copies which offer functions for shallow (copy.copy()) and deep (copy. deepcopy ()) copies. In this article, we will explore the main difference between Deep Copy and Shallow Copy.
Deep copy in Python
- A deep copy creates a new compound object before inserting copies of the items found in the original into it in a recursive manner.
- It will first construct a new collection object and then recursively populate it with copies of the child objects found in the original. It means that any changes made to a copy of the object do not reflect in the original object.
Syntax of Python Deepcopy
copy.deepcopy()
Example:
import copy
a = [[1, 2, 3], [4, 5, 6]]
# Creating a deep copy of the nested list 'a'
b = copy.deepcopy(a)
# Modifying an element in the deep-copied list
b[0][0] = 99
print(b)
import copy
a = [[1, 2, 3], [4, 5, 6]]
# Creating a deep copy of the nested list 'a'
b = copy.deepcopy(a)
# Modifying an element in the deep-copied list
b[0][0] = 99
print(b)
Output
[[99, 2, 3], [4, 5, 6]]
Explanation:
- copy.deepcopy() method creates a completely independent copy of the original object, including all nested elements. Changes made to deep_copied do not affect the original list a.
- nested elements of the original list a are recursively duplicated to ensure that even deeply nested objects are entirely independent in the copied list.
Shallow copy in Python
- A shallow copy creates a new object but retains references to the objects contained within the original. It only copies the top-level structure without duplicating nested elements.
- Changes made to a copy of an object do reflect in the original object. In python, this is implemented using the "copy.copy()" function.
Syntax of Python Shallowcopy
copy.copy()
Example:
import copy
a = [[1, 2, 3], [4, 5, 6]]
# Creating a shallow copy of the nested list 'original'
b = copy.copy(a)
# Modifying an element in the shallow-copied list
b[0][0] = 99
# Printing the original and shallow-copied lists
print(b)
import copy
a = [[1, 2, 3], [4, 5, 6]]
# Creating a shallow copy of the nested list 'original'
b = copy.copy(a)
# Modifying an element in the shallow-copied list
b[0][0] = 99
# Printing the original and shallow-copied lists
print(b)
Output
[[99, 2, 3], [4, 5, 6]]
Explanation:
- A shallow copy only copies the outer structure, retaining references to the nested objects. In this case, the inner lists are shared between original and shallow_copied.
- As a result, changes to the nested elements in shallow_copied (e.g., modifying shallow_copied[0][0]) are also reflected in original.
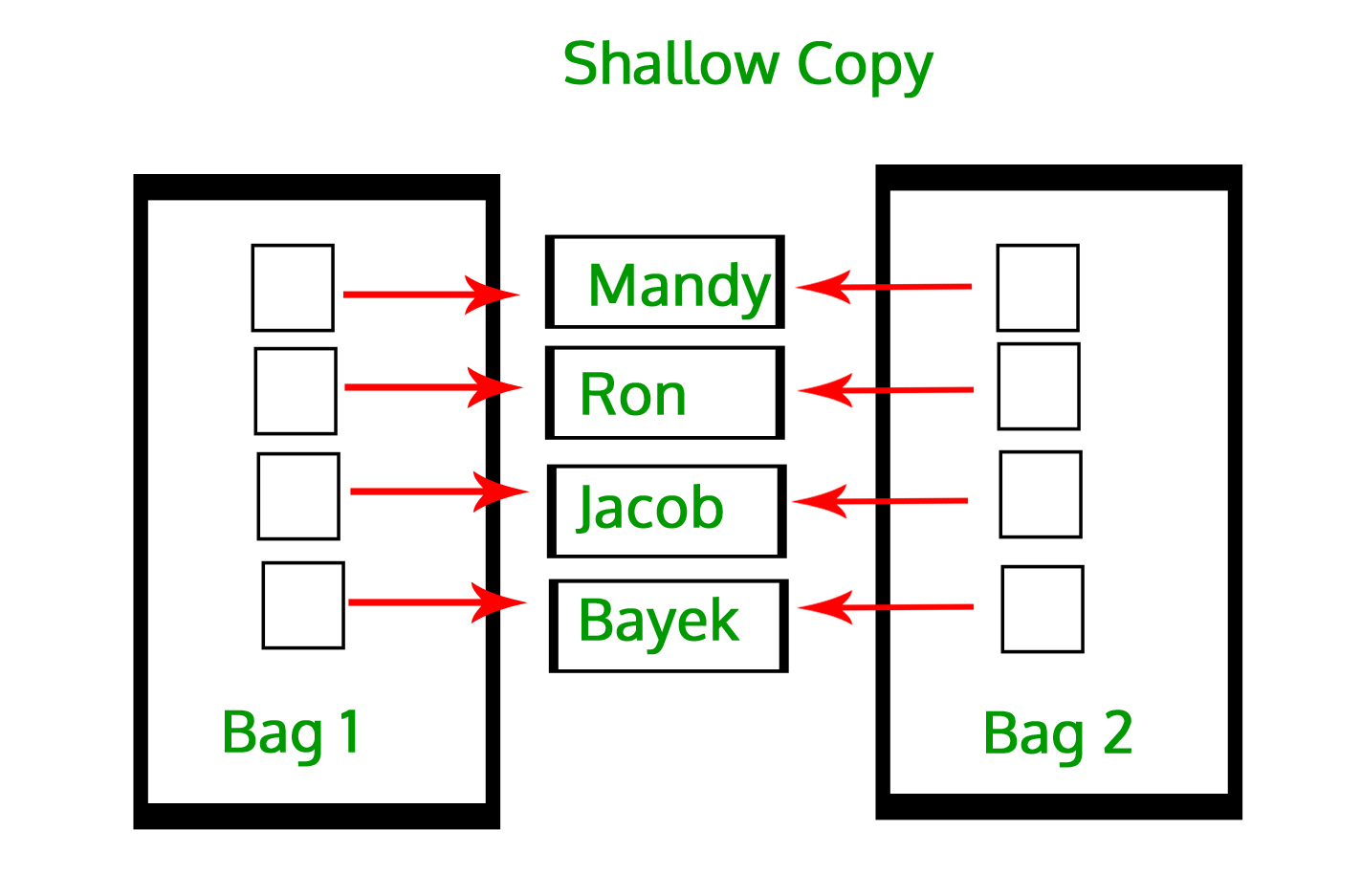
copy in Python (Deep Copy and Shallow Copy)