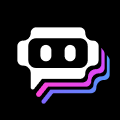
To echo (or display) an image using PHP, you typically need to set the correct headers and then output the image data. Here’s a step-by-step guide on how to do this:
Step 1: Create a PHP Script
Create a PHP file (e.g., display_image.php
) that will handle the image output.
Step 2: Set the Correct Headers
At the beginning of your PHP script, set the appropriate content type header based on the image format (e.g., JPEG, PNG, GIF).
Step 3: Read and Output the Image
Use PHP functions to read the image file and output it. Here’s an example for a JPEG image:
- <?php
- // Specify the path to the image
- $imagePath
To echo (or display) an image using PHP, you typically need to set the correct headers and then output the image data. Here’s a step-by-step guide on how to do this:
Step 1: Create a PHP Script
Create a PHP file (e.g., display_image.php
) that will handle the image output.
Step 2: Set the Correct Headers
At the beginning of your PHP script, set the appropriate content type header based on the image format (e.g., JPEG, PNG, GIF).
Step 3: Read and Output the Image
Use PHP functions to read the image file and output it. Here’s an example for a JPEG image:
- <?php
- // Specify the path to the image
- $imagePath = 'path/to/your/image.jpg';
- // Check if the image exists
- if (file_exists($imagePath)) {
- // Set the content type header for the image
- header('Content-Type: image/jpeg');
- // Read the image file and output it
- readfile($imagePath);
- exit; // Exit to prevent any additional output
- } else {
- // Handle the case where the image does not exist
- header('HTTP/1.0 404 Not Found');
- echo 'Image not found.';
- }
- ?>
Step 4: Access the Script
To display the image, you would access the display_image.php
script in your web browser. For example, if your server is running locally, you might go to:
http://localhost/display_image.php
Notes
- Make sure the path to the image is correct and that the web server has permission to read the file.
- You can change the
Content-Type
header according to the image format you are using (e.g.,image/png
,image/gif
, etc.). - This method is useful for dynamically serving images in applications, such as user uploads, where you may not want to expose the file path directly.
This approach allows you to control how images are served to users while leveraging PHP's ability to handle files and output data.
There are 2 ways, the first is straight forward & it might be what you’re looking for. You can just echo the url into an image tag?
- <?php
- $imgUrl = "myimage.jpg";
- ?>
- <img src="<?= $imgUrl; ?>"/>
straight forward.
But if what your question asks, and you want to “echo an image”, you can use headers to set the mime-type / content-type as an image.
Here is a quick snippet in PHP,
- <?php
- header('content-type: image/png');
- $theImage = "myimage.png";//the real image url.
- echo file_get_contents($theImage);
- ?>
Now, lets say the page above is “image.php”, when using an image tag, you can put “https://…/image.php” (https://…/image.php”) to
There are 2 ways, the first is straight forward & it might be what you’re looking for. You can just echo the url into an image tag?
- <?php
- $imgUrl = "myimage.jpg";
- ?>
- <img src="<?= $imgUrl; ?>"/>
straight forward.
But if what your question asks, and you want to “echo an image”, you can use headers to set the mime-type / content-type as an image.
Here is a quick snippet in PHP,
- <?php
- header('content-type: image/png');
- $theImage = "myimage.png";//the real image url.
- echo file_get_contents($theImage);
- ?>
Now, lets say the page above is “image.php”, when using an image tag, you can put “https://…/image.php” (https://…/image.php”) to display the image, the same way as “myimage.png” would.
- <img src="https://../image.php"/>
With today’s modern day tools there can be an overwhelming amount of tools to choose from to build your own website. It’s important to keep in mind these considerations when deciding on which is the right fit for you including ease of use, SEO controls, high performance hosting, flexible content management tools and scalability. Webflow allows you to build with the power of code — without writing any.
You can take control of HTML5, CSS3, and JavaScript in a completely visual canvas — and let Webflow translate your design into clean, semantic code that’s ready to publish to the web, or hand off
With today’s modern day tools there can be an overwhelming amount of tools to choose from to build your own website. It’s important to keep in mind these considerations when deciding on which is the right fit for you including ease of use, SEO controls, high performance hosting, flexible content management tools and scalability. Webflow allows you to build with the power of code — without writing any.
You can take control of HTML5, CSS3, and JavaScript in a completely visual canvas — and let Webflow translate your design into clean, semantic code that’s ready to publish to the web, or hand off to developers.
If you prefer more customization you can also expand the power of Webflow by adding custom code on the page, in the <head>, or before the </head> of any page.
Trusted by over 60,000+ freelancers and agencies, explore Webflow features including:
- Designer: The power of CSS, HTML, and Javascript in a visual canvas.
- CMS: Define your own content structure, and design with real data.
- Interactions: Build websites interactions and animations visually.
- SEO: Optimize your website with controls, hosting and flexible tools.
- Hosting: Set up lightning-fast managed hosting in just a few clicks.
- Grid: Build smart, responsive, CSS grid-powered layouts in Webflow visually.
Discover why our global customers love and use Webflow.com | Create a custom website.
1. Open an image file as a binary type.
2. Get the file contents.
3. Change content type to image accordingly.
4. echo contents.
- <?php
- $filename = "/path/to/your/file.jpg";
- $handle = fopen($filename, "rb");
- $contents = fread($handle, filesize($filename));
- fclose($handle);
- header("content-type: image/jpeg");
- echo $contents;
- ?>
try this code
- <?PHP
- $filepath= 'folder\file.jpeg';
- echo '<img src="'.$filepath.'">';
- ?>
or
- <?PHP
- $filepath= '\folder\file.jpeg';
- echo '<img src="'.$filepath.'">';
- ?>
As with the first answer, I'm not sure what you're trying to achieve. PHP is a server side interpretive language used to produce html. With this in mind, (I'm guessing) what you're after is the same way that html "shows" an image; using the img tag. ie. html would simply be
<img src="smiley.gif" alt="Smiley face" height="42" width="42">
This can be achieved in PHP with;
echo '
<img src="smiley.gif" alt="Smiley face" height="42" width="42">';
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of th
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of the biggest mistakes and easiest ones to fix.
Overpaying on car insurance
You’ve heard it a million times before, but the average American family still overspends by $417/year on car insurance.
If you’ve been with the same insurer for years, chances are you are one of them.
Pull up Coverage.com, a free site that will compare prices for you, answer the questions on the page, and it will show you how much you could be saving.
That’s it. You’ll likely be saving a bunch of money. Here’s a link to give it a try.
Consistently being in debt
If you’ve got $10K+ in debt (credit cards…medical bills…anything really) you could use a debt relief program and potentially reduce by over 20%.
Here’s how to see if you qualify:
Head over to this Debt Relief comparison website here, then simply answer the questions to see if you qualify.
It’s as simple as that. You’ll likely end up paying less than you owed before and you could be debt free in as little as 2 years.
Missing out on free money to invest
It’s no secret that millionaires love investing, but for the rest of us, it can seem out of reach.
Times have changed. There are a number of investing platforms that will give you a bonus to open an account and get started. All you have to do is open the account and invest at least $25, and you could get up to $1000 in bonus.
Pretty sweet deal right? Here is a link to some of the best options.
Having bad credit
A low credit score can come back to bite you in so many ways in the future.
From that next rental application to getting approved for any type of loan or credit card, if you have a bad history with credit, the good news is you can fix it.
Head over to BankRate.com and answer a few questions to see if you qualify. It only takes a few minutes and could save you from a major upset down the line.
How to get started
Hope this helps! Here are the links to get started:
Have a separate savings account
Stop overpaying for car insurance
Finally get out of debt
Start investing with a free bonus
Fix your credit
For sake of this example, “Oregon-Coast.jpg” will be the file name. Now then, let’s echo it. Here is a code snippet.
- $pic = “Oregon-Coast.jpg”;
- echo “<img src=‘”.$pic.“’>”;
- <?PHP
- $filepath= ‘/folder/file.jpeg’;
- echo ‘<img src=“’.$filepath.‘”>'
- ?>
Remember basic HTML structure while coding in PHP
The reason you should hire a digital marketing freelancer is that it can be very overwhelming trying to do this on your own–which is why so many people and businesses outsource that work. Fiverr freelancers offer incredible value and expertise and will take your digital marketing from creation to transaction. Their talented freelancers can provide full web creation or anything Shopify on your budget and deadline. Hire a digital marketing freelancer on Fiverr and get the most out of your website today.
echo '<img src="<FILE_PATH>"/>';
echo takes string as parameter,I'm passing image tag of html. So browser will interpret that tag and will display an image
- <?php
- $imgData = base64_encode(file_get_contents('/folder/file.jpeg'));
- $src = 'data: '.mime_content_type('/folder/file.jpeg').';base64,'.$imgData;
- echo "<img src=\"$src\" >";
- //Step 1:we read the image file and convert it to text encoded format
- //Step 2: we prepare the data for <img> src tag to display the text //encoded format
- // We write the <img> tag with the data for the browser to display
- It's just like the normal img tag with the source of the file. The only difference here is that since you are using php, you have to use the echo statement. Example is echo "<img src =’imageaddress>
If you want to echo image from file just use below code
echo '<img src="file path with extension"/>';
If you are retrieving image from database or it is in binary format you have to set header
header("content-type:image/jpeg");
echo $image;
Here $image contains the binary string of source image.
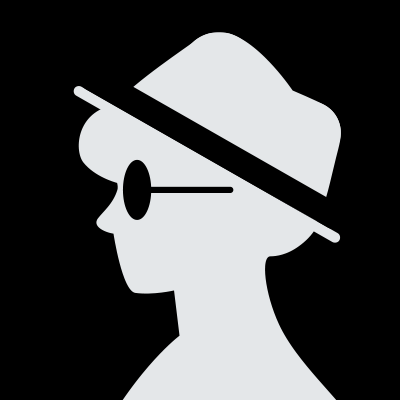
I am not sure what you want to achieve but what you can do is to echo the url of an image.
You can also use array and foreach loop to echo multiple images.
You can check out the code snippet here.
Here is some code to echo a 1x1 pixel PNG image:
// 1x1 PNG image
$image = base64_decode('iVBORw0KGgoAAAANSUhEUgAAAAEAAAABAQMAAAAl21bKAAAAA1BMVEUAAACnej3aAAAAAXRSTlMAQObYZgAAAApJREFUCNdjYAAAAAIAAeIhvDMAAAAASUVORK5CYII=');
header('Content-Type: image/png');
echo $image;
Don’t echo it. Close the PHP where you want the ‘echo’, then use html and open the php up again.
php only echos an image's path:
<img src="<?php echo 'http://google.com/images/abc.jpg'; ?>" />
If you are trying to echo an image that is retrieved via a DB call, then you need to echo both the image and the appropriate header
- $sql="select image from $table where image_id=1";
- $result =mysqli_query($sql);
- $image = mysqli_result($result, 0, 'image');
- header('Content-Type: image/jpeg');Echo $image;
I honestly can’t think of a single instance I would want to.
The only practical difference I can think of is that print() operates like a function that always returns 1.
You could in theory:
- true && true && print ('hello');
Outputs:
- hello
The following:
- true && false && print ('hello');
Outputs:
Yup, nothing.
Let’s try this with echo.
The following:
- true && false && echo ('hello');
Outputs:
- PHP Parse error: syntax error, unexpected 'echo' (T_ECHO) in test.php on line 2
Oh dear!
So let’s say I wanted to do a print out something if an IF statement is positive:
- $i = 0;
- if (true && true && print ('added')) {
- $i +
I honestly can’t think of a single instance I would want to.
The only practical difference I can think of is that print() operates like a function that always returns 1.
You could in theory:
- true && true && print ('hello');
Outputs:
- hello
The following:
- true && false && print ('hello');
Outputs:
Yup, nothing.
Let’s try this with echo.
The following:
- true && false && echo ('hello');
Outputs:
- PHP Parse error: syntax error, unexpected 'echo' (T_ECHO) in test.php on line 2
Oh dear!
So let’s say I wanted to do a print out something if an IF statement is positive:
- $i = 0;
- if (true && true && print ('added')) {
- $i += 1;
- }
Outputs:
- added
I would find the following easier to read
- $i = 0;
- if (true && true) {
- echo 'added';
- $i += 1;
- }
Conclusion
It does have it’s unique qualities, but I can’t think of a single place I’d actually use it. It would make the code less readable at the savings of only a few characters.
Readability is king in programming.
After Thought
Maybe there is 1 case in debugging a long if statement I could think of:
- $a = 1;
- $b = 1;
- $c = 1;
- $d = 1;
- $e = 1;
- if ($a && $b && $c && $d && $e) {
- echo 'User Signed In';
- }
In the above code, how can you easily debug which is failing?
- $a = 1;
- $b = 1;
- $c = 1;
- $d = 1;
- $e = 1;
- if ($a && print ("Got Past a\n") &&
- $b && print ("Got Past b\n") &&
- $c && print ("Got Past c\n") &&
- $d && print ("Got Past d\n") &&
- $e && print ("Got Past e\n")
- ) {
- echo 'User Signed In';
- }
Unfortunately, this still doesn’t work as I would have expected, I get the output:
- Got Past e
- 1111User Signed In
After after thought
Just stick with echo, it’s also just 1 character less to be typed.
This is a pretty basic question so I apologize if my answer gets too simplistic.
For where you are at, you can think of a PHP file as just an HTML file that lets you occasionally interrupt the HTML layout to do something in PHP.
So if this is the HTML for a page with a single image:
- <!DOCTYPE html>
- <html lang='en'>
- <head>
- <title>Test Page</title>
- </head>
- <body>
- <img src='path/to/myphoto.jpg' alt='photo of me' />
- </body>
- </html>
Then this is that same page with a little PHP to output the HTML image tag:
- <!DOCTYPE html>
- <html lang='en'>
- <head>
- <title>Test Page</title>
- </head>
- <body>
- <?php
- echo "<img
This is a pretty basic question so I apologize if my answer gets too simplistic.
For where you are at, you can think of a PHP file as just an HTML file that lets you occasionally interrupt the HTML layout to do something in PHP.
So if this is the HTML for a page with a single image:
- <!DOCTYPE html>
- <html lang='en'>
- <head>
- <title>Test Page</title>
- </head>
- <body>
- <img src='path/to/myphoto.jpg' alt='photo of me' />
- </body>
- </html>
Then this is that same page with a little PHP to output the HTML image tag:
- <!DOCTYPE html>
- <html lang='en'>
- <head>
- <title>Test Page</title>
- </head>
- <body>
- <?php
- echo "<img src='path/to/myphoto.jpg' alt='photo of me' />";
- ?>
- </body>
- </html>
The PHP code starts where you see <?php and ends where you see ?>.
Just to expand the example a little, let’s say you wanted a PHP page to randomly show a picture of either you or your dog on each page load. That might look something like this:
- <!DOCTYPE html>
- <html lang='en'>
- <head>
- <title>Test Page</title>
- </head>
- <body>
- <?php
- // Randomly assign either a 0 or 1 to the variable $show_my_dog.
- $show_my_dog = rand(0,1);
- if( $show_my_dog == 1 )
- echo "<img src='path/to/mydog.jpg' alt='photo of my dog' />";
- else
- echo "<img src='path/to/myphoto.jpg' alt='photo of me' />";
- ?>
- </body>
- </html>
Hope that helps get you started!
for Inserting image
CODE:
<html>
<head><title>Upload - photo</title>
<style>
.up_pho
{
border:1px solid #BEBEBE;
margin:50px 400px 40px 40px;
padding:10px 10px 10px 10px;
}
</style>
</head>
<body>
<div class=up_pho ><?php
$rollno = rollno;
?>
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method=post enctype="multipart/form-data" >
<input type=hidden name="MAX_FILE_SIZE" value="1000000" />
<input type="file" name="userfile">
<input type=submit value=submit />
</form>
<?php
// Checking the file was submitted
if(!isset($_FILES['userfile'])) { echo '<p>Please Select a file</p>'; }
else
for Inserting image
CODE:
<html>
<head><title>Upload - photo</title>
<style>
.up_pho
{
border:1px solid #BEBEBE;
margin:50px 400px 40px 40px;
padding:10px 10px 10px 10px;
}
</style>
</head>
<body>
<div class=up_pho ><?php
$rollno = rollno;
?>
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method=post enctype="multipart/form-data" >
<input type=hidden name="MAX_FILE_SIZE" value="1000000" />
<input type="file" name="userfile">
<input type=submit value=submit />
</form>
<?php
// Checking the file was submitted
if(!isset($_FILES['userfile'])) { echo '<p>Please Select a file</p>'; }
else
{ try {
$msg = upload(); // function calling to upload an image
echo $msg;
}
catch(Exception $e) {
echo $e->getMessage();
echo 'Sorry, Could not upload file';
}
}
function upload() {
//include "database/dbco.php";
$maxsize = 10000000; //set to approx 10 MB
//check associated error code
if($_FILES['userfile']['error']==UPLOAD_ERR_OK) {
//check whether file is uploaded with HTTP POST
if(is_uploaded_file($_FILES['userfile']['tmp_name'])) {
//checks size of uploaded image on server side
if( $_FILES['userfile']['size'] < $maxsize) {
//checks whether uploaded file is of image type
if($_FILES['userfile']['type']=="image/gif" || $_FILES['userfile']['type']== "image/png" || $_FILES['userfile']['type']== "image/jpeg" || $_FILES['userfile']['type']== "image/JPEG" || $_FILES['userfile']['type']== "image/PNG" || $_FILES['userfile']['type']== "image/GIF") {
// prepare the image for insertion
$imgData =addslashes (file_get_contents($_FILES['userfile']['tmp_name']));
// put the image in the db...
// database connection
$host = 'localhost';
$user = 'root';
$pass = '';
$db = 'image';
mysql_connect($host, $user, $pass) OR DIE (mysql_error());
// select the db
mysql_select_db ($db) OR DIE ("Unable to select db".mysql_error());
// our sql query
$sql = "INSERT INTO storeimages
(id,image, name,size)
VALUES
('$_POST[id]','{$imgData}', '{$_FILES['userfile']['name']}','{$_FILES['userfile']['size']}');";
mysql_query($sql) or die("Error in Query insert: " . mysql_error());
// insert the image
$msg='<p>Image successfully saved in database . </p>';
}
else
$msg="<p>Uploaded file is not an image.</p>";
}
else {
// if the file is not less than the maximum allowed, print an error
$msg='<div>File exceeds the Maximum File limit</div>
<div>Maximum File limit is '.$maxsize.' bytes</div>
<div>File '.$_FILES['userfile']['name'].' is '.$_FILES['userfile']['size'].
' bytes</div><hr />';
}
}
else
$msg="File not uploaded successfully.";
}
else {
$msg= file_upload_error_message($_FILES['userfile']['error']);
}
return $msg;
}
// Function to return error message based on error code
function file_upload_error_message($error_code) {
switch ($error_code) {
case UPLOAD_ERR_INI_SIZE:
return 'The uploaded file exceeds the upload_max_filesize directive in php.ini';
case UPLOAD_ERR_FORM_SIZE:
return 'The uploaded file exceeds the MAX_FILE_SIZE directive that was specified in the HTML form';
case UPLOAD_ERR_PARTIAL:
return 'The uploaded file was only partially uploaded';
case UPLOAD_ERR_NO_FILE:
return 'No file was uploaded';
case UPLOAD_ERR_NO_TMP_DIR:
return 'Missing a temporary folder';
case UPLOAD_ERR_CANT_WRITE:
return 'Failed to write file to disk';
case UPLOAD_ERR_EXTENSION:
return 'File upload stopped by extension';
default:
return 'Unknown upload error';
}
}
?>
</div>
</body>
</html>
CODE FOR RETRIEVING IMAGE
<?php
// Store File in a name : DisplayPhoto.php
$host = 'localhost';
$user = 'root';
$pass = '';
$db = 'image';
// some basic sanity checks
if(isset($_POST['id']) && is_numeric($_POST['id'])) {
//connect to the db
$link = mysql_connect("$host", "$user", "$pass")
or die("Could not connect: " . mysql_error());
// select our database
mysql_select_db("$db") or die(mysql_error());
// get the image from the db
$sql = "SELECT image FROM storeimages WHERE id=" .$_POST['id'] . ";";
// the result of the query
$result = mysql_query("$sql") or die("Invalid query: " . mysql_error());
// set the header for the image
header("Content-type: image/jpeg");
echo mysql_result($result, 0);
// close the db link
mysql_close($link);
}
else {
echo 'Please use a real id number';
}
?>
In PHP ; both ‘echo’ and ‘print’ are quite similar but there are bit differences.
- One of the difference is ‘echo’ does not have any return value but ‘print’ has return value =1; if it success. So for that cause the execution time of program is naturally faster using ‘echo’ instead of ‘print’.
- Another difference is you cant print multiple arguments separated by comma using ‘print’ . Follow below codes to better understand-
- <?php
- echo "I am here<br>" , "with you";
- print "<br>I am here";
- ?>
- In above coding you cant get any syntax error .
Now again follow below code-
- <?php
- echo "I am here<br>", "with you";
- p
In PHP ; both ‘echo’ and ‘print’ are quite similar but there are bit differences.
- One of the difference is ‘echo’ does not have any return value but ‘print’ has return value =1; if it success. So for that cause the execution time of program is naturally faster using ‘echo’ instead of ‘print’.
- Another difference is you cant print multiple arguments separated by comma using ‘print’ . Follow below codes to better understand-
- <?php
- echo "I am here<br>" , "with you";
- print "<br>I am here";
- ?>
- In above coding you cant get any syntax error .
Now again follow below code-
- <?php
- echo "I am here<br>", "with you";
- print "<br>I am here", "with you";
- ?>
- Now here you will definitely get syntax error for using multiple arguments separated by comma using ‘print’.
So those are two main differences . That is why might be all PHP developers use echo instead of print.
echo and print both are PHP Statement are used to display the output in PHP.
echo
- echo is a statement i.e used to display the output. it can be used with parentheses echo or without parentheses echo.
- echo can pass multiple strings separated as ( , )
- echo doesn’t return any value
- echo is faster than print
- eho is not a function but a language construct.
- print is also a statement i.e used to display the output. it can be used with parentheses print( ) or without parentheses print.
- using print can doesn’t pass multiple argument
- print always return 1
- it is slower than echo
- In PHP, print is not a real fun
echo and print both are PHP Statement are used to display the output in PHP.
echo
- echo is a statement i.e used to display the output. it can be used with parentheses echo or without parentheses echo.
- echo can pass multiple strings separated as ( , )
- echo doesn’t return any value
- echo is faster than print
- eho is not a function but a language construct.
- print is also a statement i.e used to display the output. it can be used with parentheses print( ) or without parentheses print.
- using print can doesn’t pass multiple argument
- print always return 1
- it is slower than echo
- In PHP, print is not a real function but a language construct. However, it behaves like a function in that it returns a value.
- It can be used in expressions.
Yes, echo is the more commonly used form of putting data on the screen. But you want to be careful when you use it that the data being output is sanitary. Meaning something that can't break the environment it's running in. Example a web page if you echo HTML that is based on a users input the I can use that input to create an elaborate page within your page and potentially hijack your site then eventually maybe even your server santized means you are treating everything a user can input as hostile and are using HTML entities and encoding and other methods to scrub data clean to prevent it from
Yes, echo is the more commonly used form of putting data on the screen. But you want to be careful when you use it that the data being output is sanitary. Meaning something that can't break the environment it's running in. Example a web page if you echo HTML that is based on a users input the I can use that input to create an elaborate page within your page and potentially hijack your site then eventually maybe even your server santized means you are treating everything a user can input as hostile and are using HTML entities and encoding and other methods to scrub data clean to prevent it from breaking through some other unintended means
The obvious answer is print_r (or var_dump) as answered by many people. But take this tip from a ~17-year PHP geek. The problem with the output from print_r or var_dump is it is not re-usable in any way. You can’t copy/paste the output into a variable definition and expect to be able to re-use it. When I’m quickly hacking through things, sure, I’ll echo things. But over the years I’ve converted and anything more complex I’ve gotten in the habit of instead using json_encode instead of print_r. And if you want it to look sexy, use the pretty print option.
Here, let me show you how and why…
- $arr =
The obvious answer is print_r (or var_dump) as answered by many people. But take this tip from a ~17-year PHP geek. The problem with the output from print_r or var_dump is it is not re-usable in any way. You can’t copy/paste the output into a variable definition and expect to be able to re-use it. When I’m quickly hacking through things, sure, I’ll echo things. But over the years I’ve converted and anything more complex I’ve gotten in the habit of instead using json_encode instead of print_r. And if you want it to look sexy, use the pretty print option.
Here, let me show you how and why…
- $arr = array('one', 'two', 'three');
- echo json_encode($arr, JSON_PRETTY_PRINT);
Will output…
- [
- "one",
- "two",
- "three"
- ]
Which you can copy/paste right back into PHP to use. For example…
- $arr = json_decode([
- "one",
- "two",
- "three"
- ]);
- print_r($arr);
Will output…
- Array
- (
- [0] => one
- [1] => two
- [2] => three
- )
This is in my opinion, far superior to print_r/var_dump, as you’ll be able to for example, take the output of a function, and re-use it over and over for debugging the next step in the logical flow of an application when you’re stepping through your code. Take it from an hardcore php veteran. :)
PLEASE NOTE: This does not work on OBJECTs, for that you should use PHP’s serialize() so you can unserialize them, but then they become _nearly_ unreadable again. But if you know your data is an array or simple object and you don’t care about being able to replicate it, then it’s okay to json encode it. :)
Cheers!
Literally displays whatever text you tell it to. To get more complicated... It will also echo the type if you give it an array (unless they changed it).
Google print vs echo for some more details, as they do have their limitations. And the speed depends on the type of variable you give the function.
Before checking the difference between these two, lets look into little deep.
echo
and print()
are language constructs .
That means the php compiler knows the exact syntax of the echo/print()
statement. Unlike built in functions, language constructors act like some keywords, they already defined by the compiler.
Other main advantage of language constructor over built in function is, it’s little more faster than built in functions.
Now lets come to the answer, both these statements are used to print a value.
Main differences are
echo
do not return anything whileprint()
return a value(1)
- $var a = echo
Before checking the difference between these two, lets look into little deep.
echo
and print()
are language constructs .
That means the php compiler knows the exact syntax of the echo/print()
statement. Unlike built in functions, language constructors act like some keywords, they already defined by the compiler.
Other main advantage of language constructor over built in function is, it’s little more faster than built in functions.
Now lets come to the answer, both these statements are used to print a value.
Main differences are
echo
do not return anything whileprint()
return a value(1)
- $var a = echo “something”; //return error since echo return no value;
- $var b = print(“somethning”); //return 1
- Multiple values passed to echo, but only one value can pass to print()
- echo “something”,100,3.14,’A’;
- print(“something”,1,3.14); //shows error
- Echo is much more faster than
print()
- Speed: There is a difference between the two, but speed-wise it should be irrelevant which one you use. echo is marginally faster since it doesn't set a return value if you really want to get down to the nitty gritty.
- Expression:
print()
behaves like a function in that you can do:$ret = print "Hello World"
; And$ret
will be1
. That means that print can be used as part of a more complex expression where echo cannot. An example from the PHP Manual:
- $b ? print "true" : print "false";
- print is also part of the precedence table which it needs to be if it is to be used within a complex expression. It
- Speed: There is a difference between the two, but speed-wise it should be irrelevant which one you use. echo is marginally faster since it doesn't set a return value if you really want to get down to the nitty gritty.
- Expression:
print()
behaves like a function in that you can do:$ret = print "Hello World"
; And$ret
will be1
. That means that print can be used as part of a more complex expression where echo cannot. An example from the PHP Manual:
- $b ? print "true" : print "false";
- print is also part of the precedence table which it needs to be if it is to be used within a complex expression. It is just about at the bottom of the precedence list though. Only "," AND, OR and XOR are lower.
- Parameter(s): The grammar is:
echo expression [, expression[, expression] ... ]
Butecho ( expression, expression )
is not valid. This would be valid:echo ("howdy"),("partner")
; the same as:echo "howdy","partner"
; (Putting the brackets in that simple example serves no purpose since there is no operator precedence issue with a single term like that.)
So, echo without parentheses can take multiple parameters, which get concatenated:
- echo "and a ", 1, 2, 3; // comma-separated without parentheses
- echo ("and a 123"); // just one parameter with parentheses
print()
can only take one parameter:
- print ("and a 123");
- print "and a 123";
Source : How are echo and print different in PHP?
Hope this helps :)
Keshav Khatri
With PhotoShop, click save as. There will be a long list of options with PNG being one of them. Take note of the save location or change it to wherever you want. (this is how it’s done on a Mac, Windows I don’t know)
print
and echo
are really based on preference. I prefer to use print
because majority of programming languages use print
. The only one that comes to mind that uses echo
is Bash. So I prefer using print
because I don’t have to switch between print
and echo
when I use multiple different languages. I can just simply use 1 c...
You can insert an image in PHP file by using HTML tags. Syntax is similar to HTML you can use echo to print the image but the total HTML must be present inside the PHP tags.
I stated two ways to do this work any of the two Will work but make sure when should you use the particular syntax, you Will understand this only if you know the use case of each of them.
You can do this by simply using image tag in php
- echo ' <img src="php.png" /> ';
You can also do this by inserting html tags inside
<?php and ?>
- <div>
- <img src="myPic.jpg" alt="myPic" />
- </div>
both are more or less the same. The main difference is that print has a return value of 1 so you can use it in expressions. This is also the reason why echo is faster (no return value)
Mostly people use echo because:
echo is marginally faster than print.
echo has no return value while print always returns 1 if successful.
echo can take multiple parameters (although such usage is rare) while print takes one argument.
In PHP echo simply output whatever is passed to it as parameter.
echo 'hello world' //It will show hello world
echo 'hello $name' // it will show hello Jon if $name=Jon
Also note, PHP echo is not actually considered as function. Please read PHP: echo - Manual for details
This works, and can be called a right way but there are other ways for as and when the situation may demand.
For example,
You can break the php code like this:
- <?php if(condition){ ?>
- <!-- HTML here -->
- <?php } ?>
But this is more suitable for displaying larger HTML content. Can also be used without the conditional statement.
Alternatively, you may utilize the heredoc syntax supported in php5+, as follows:
- echo <<<ABC
- This can be any HTML or general string content with variable interpolation
- ABC;
Here, ABC can be any random characters. But make sure to NOT indent the closing 'ABC;' and NO whit
This works, and can be called a right way but there are other ways for as and when the situation may demand.
For example,
You can break the php code like this:
- <?php if(condition){ ?>
- <!-- HTML here -->
- <?php } ?>
But this is more suitable for displaying larger HTML content. Can also be used without the conditional statement.
Alternatively, you may utilize the heredoc syntax supported in php5+, as follows:
- echo <<<ABC
- This can be any HTML or general string content with variable interpolation
- ABC;
Here, ABC can be any random characters. But make sure to NOT indent the closing 'ABC;' and NO whitespaces preceding the semi-colon too.
Or, you can just assign the html string to a variable and then echo the variable.
There is no one "right" way. :)
Depends on what you mean by "add elements to a web site". If your simply adding a div, the correct way to add it in a PHP website is the same way you add it in a raw HTML website. That is, <div></div>. If however, you are building something dynamic, or something that requires back-end data, then you may desire other options. In this case
echo '<div></div>';
would be perfectly acceptable
as in
- $file_list = FileManager::getListofReports();
- foreach ($file_list as $file) {
- echo '<div><a target="_blank" href="echo $file">echo $file</a></div>';
- }
or, a combination of both, as in:
- $file_list = FileMan
Depends on what you mean by "add elements to a web site". If your simply adding a div, the correct way to add it in a PHP website is the same way you add it in a raw HTML website. That is, <div></div>. If however, you are building something dynamic, or something that requires back-end data, then you may desire other options. In this case
echo '<div></div>';
would be perfectly acceptable
as in
- $file_list = FileManager::getListofReports();
- foreach ($file_list as $file) {
- echo '<div><a target="_blank" href="echo $file">echo $file</a></div>';
- }
or, a combination of both, as in:
- $file_list = FileManager::getListofReports();
- foreach ($file_list as $file) { ?>
- <div style="padding-right: 15px;">
- <a style="color: #fff;" target="_blank" href="<?php echo '$file' ?>"><?php echo $file ?>
- </a>
- </div>
- <?php } ?>
You wouldn’t scan them, but retreive them.
A simple way would be to create a page with a form:
- Enter target URL or have a bookmakrlet to do this part for you
- Retrieve the page
PHP: cURL - Manual or
file_get_contents - Manual - Parse out the images from the HTML -
PHP: DOM - Manual - Download the images using, say, curl again or even just copy
PHP: copy - Manual
A PHP script can be used with a HTML form to allow users to upload files to the server. Initially files are uploaded into a temporary directory and then relocated to a target destination by a PHP script.
Information in the phpinfo.php page describes the temporary directory that is used for file uploads as upload_tmp_dir and the maximum permitted size of files that can be uploaded is stated as upload_max_filesize. These parameters are set into PHP configuration file php.ini
The process of uploading a file follows these steps −
- The user opens the page containing a HTML form featuring a text files,
A PHP script can be used with a HTML form to allow users to upload files to the server. Initially files are uploaded into a temporary directory and then relocated to a target destination by a PHP script.
Information in the phpinfo.php page describes the temporary directory that is used for file uploads as upload_tmp_dir and the maximum permitted size of files that can be uploaded is stated as upload_max_filesize. These parameters are set into PHP configuration file php.ini
The process of uploading a file follows these steps −
- The user opens the page containing a HTML form featuring a text files, a browse button and a submit button.
- The user clicks the browse button and selects a file to upload from the local PC.
- The full path to the selected file appears in the text filed then the user clicks the submit button.
- The selected file is sent to the temporary directory on the server.
- The PHP script that was specified as the form handler in the form's action attribute checks that the file has arrived and then copies the file into an intended directory.
- The PHP script confirms the success to the user.
As usual when writing files it is necessary for both temporary and final locations to have permissions set that enable file writing. If either is set to be read-only then process will fail.
An uploaded file could be a text file or image file or any document.
Creating an upload form
The following HTM code below creates an uploader form. This form is having method attribute set to post and enctype attribute is set to multipart/form-data
- <?php
- if(isset($_FILES['image'])){
- $errors= array();
- $file_name = $_FILES['image']['name'];
- $file_size =$_FILES['image']['size'];
- $file_tmp =$_FILES['image']['tmp_name'];
- $file_type=$_FILES['image']['type'];
- $file_ext=strtolower(end(explode('.',$_FILES['image']['name'])));
- $extensions= array("jpeg","jpg","png");
- if(in_array($file_ext,$extensions)=== false){
- $errors[]="extension not allowed, please choose a JPEG or PNG file.";
- }
- if($file_size > 2097152){
- $errors[]='File size must be excately 2 MB';
- }
- if(empty($errors)==true){
- move_uploaded_file($file_tmp,"images/".$file_name);
- echo "Success";
- }else{
- print_r($errors);
- }
- }
- ?>
- <html>
- <body>
- <form action="" method="POST" enctype="multipart/form-data">
- <input type="file" name="image" />
- <input type="submit"/>
- </form>
- </body>
- </html>
It will produce the following result −
You don’t do it directly with PHP. PHP is a server side language.
You need to use PHP to generate the HTML and send it to the browser.
The image itself should not be in the database. That’s a very bad idea. You usually have the image on the file system. The path and the image name are what is saved in the database.
You call that up from the database, and use it to genera...
echo() and print() are not functions but language constructs in PHP. They are both used to output strings and there are very minor differences between echo and print in PHP.
Speed of reverberate versus print in PHP -
The speed of both reverberate and print articulations in PHP is generally the same. Utilizing one over the other isn't probably going to yield any execution change in your application. Hypothetically, resound is more effective in light of the fact that it doesn't restore any esteem.
Capacity versus Language Construct -
Not at all like most PHP string capacities, reverberate and print
echo() and print() are not functions but language constructs in PHP. They are both used to output strings and there are very minor differences between echo and print in PHP.
Speed of reverberate versus print in PHP -
The speed of both reverberate and print articulations in PHP is generally the same. Utilizing one over the other isn't probably going to yield any execution change in your application. Hypothetically, resound is more effective in light of the fact that it doesn't restore any esteem.
Capacity versus Language Construct -
Not at all like most PHP string capacities, reverberate and print aren't works however dialect builds. In this manner it isn't required to utilize brackets when utilizing reverberation or print.
There are several methods.
- Display entire array at once - print_r($array) ;
- Display each and every array in a loop
foreach($array as $value){
print_r($value);
}
in this step you can go further by looping your $value array
You'd need to define "send". If you mean, can I send an image to the server (upload)? Then yes, quite easily.
Can I send an image in an email by PHP? Possibly, you'd need to use an upload script to upload it, then create an email, attaching the image to the email and sending it. It is possible but difficult.
The third option would be emailing it to an address not...